Android ListView
Overview
We will learn about how to show lists of objects in an android app using the listview widget.
Pre Requisites
Finish the Android Intro project before trying this project.
Concepts
In this section, we will explain how a ListView works in Android, ListView is a widget that is used to show collections of objects (eg: List of contacts or list of countries etc). ListView is a container widget. For each item in the list, the ListView widget will contain another View that represents the item to be shown. This item view can be made up of regular widgets such as textview, imageview, buttons etc.
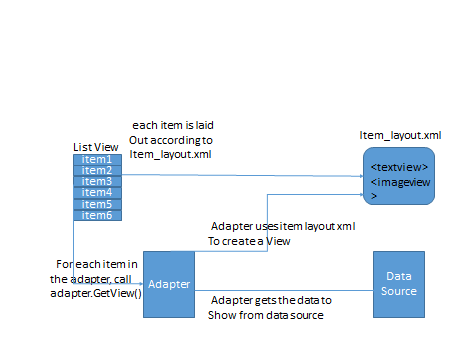
Following is the approximate flow sequence for a listview to work:
- Define an activity and place a listview in the activity's layout file.
- Define another layout file that holds the layout for the items that will be shown inside the list. This is what we refer to as the item layout xml file in the above diagram.
- Override the ArrayAdapter class (or any other adapter class) and provide your implementation for GetView() method. This method will return the View object for each item in the listview. The View returned by this method will match the layout defined in the item layout xml.
- Create an instance of your adapter class - You need to pass the id of the item layout xml file as well as the id of a textview widget contained in the item layout as arguments to the constructor of the adapter class.
- Call setAdapter on the listview to bind the listview to your adapter instance.
The Tutorial section will walk you through an example.
ListView Performance
Since lists can show thousands of data items, it is important to understand performance aspects of listview.
- Pagination, Continuous Scrolling - It is always a good idea to provide a pagination/continuous scrolling approach where only the data needed to be shown is fetched/loaded (instead of fetching and showing the entire data set). The pagination project activity will walk you through this exercise.
- Handle Row View Recycling - When a user scrolls through a list of items, android will reuse an existing row of the listview to show new data (ie it wont allocate new rows as new items are shown). This is described in more detail on codepath's github page and at software-workshop's blog. If the convertView parameter is null, then a new View needs to be called by calling inflater.Inflate(). If the convertView parameter in the call to getView is not null, then it is a view that is being recycled. You need to ensure all the properties on this reused view are set correctly to match the new data that is being bound to that view. Android source code for ArrayAdapter.getView() shows this approach nicely as shown in the snippet below:
- ViewHolder Pattern - Instead of calling findViewById for every row in the listview, you can cache the view definitions. See the ViewHolder pattern for more details.
- Use Background threads for processing (fetching data, images etc) - This will free up the main UI thread for a smoother scrolling experience. See this android developer documentation for more details.
- Implement RecyclerListener to free up resources when a view is put on scrap heap - During scrolling, when a view is no longer visible, it is recycled into a scrap heap. It can then be reused to display a new item (passed as convertView parameter in the call to getView). If the view is holding expensive resources (such as images), you can implement RecyclerListener to perform cleanup action when the view is recycled. This is explained in more detail at chess-ix blog and at Sriram Ramani's blog. In the tutorial below, the activity implements RecyclerListener to free up image resources.
Setup
Follow the Getting Started on Android document to get your environment up and running for developing Android apps
Tutorial
Lets walk through an example. Suppose you want to show a list of contacts. Each contact will have the name and an image shown.
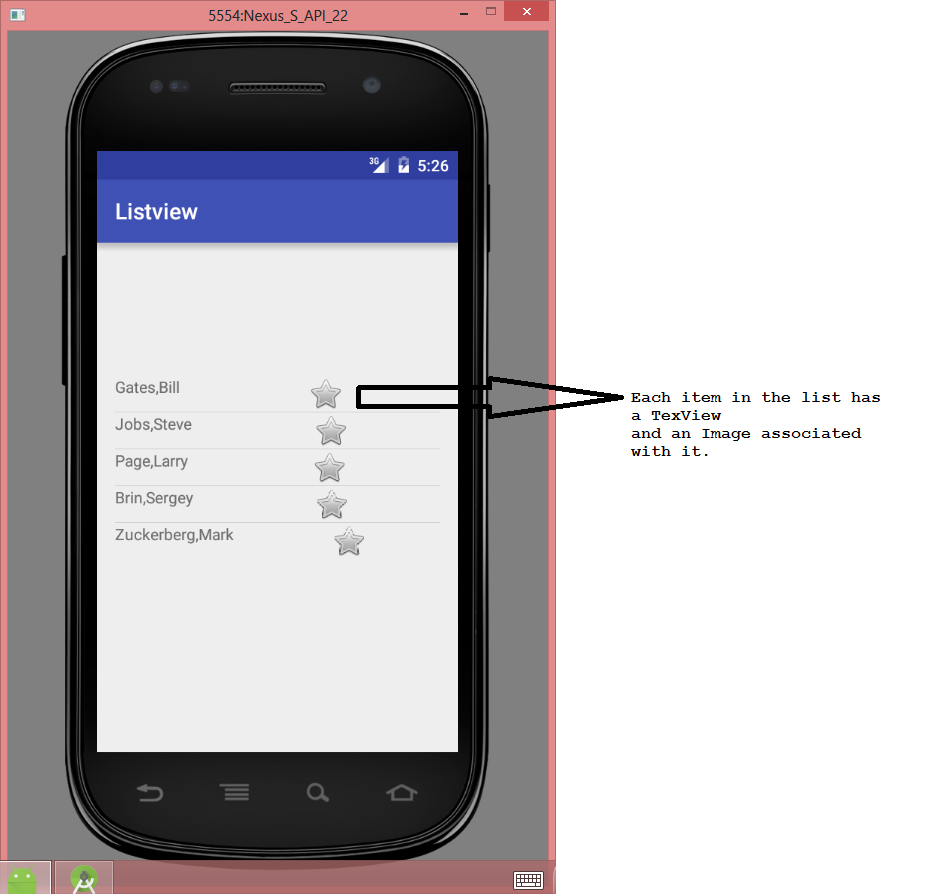
For simplicity, we are just showing a Star image next to each contact name, but you can easily extend the example to show a contact's photo for example. The following are the various components needed to build the above android app:
- Define an activity for the listview. Place only the listview widget in the activity's layout file. You can look at activity_image_view_tutorial.xml file of the project that is shown below
- Now, define a layout XML file for the items in the list view: Since we want to show contact name (Text) and an image for each item, you can create a new layout file (In Android Studio: New -> XML -> Layout XML File) and place TextView and ImageView widgets in the layout file. You can look at imageview_tutorial_layout file which is shown in the snippet below.
- Next, provide an implementation of an ArrayAdapter that overrides the default GetView() implementation and returns the above View (comprising of TextView and ImageView widgets) for every row in the list. You can either override the GetView method inline or create a new custom adapter subclass as shown in the ImageViewTutorialActivity.java file
In both implementations, we call the super.getView() method to get the View associated with the adapter and let the base ArrayAdapter handle inflating the view/handle view recycling. The code expects this to be a view with 2 widgets (TextView and ImageView). We set the value of TextView to hold the contact's FullName. The code sets the ImageView to the btn_star image resource to show a star image next to the contact.
- Notice that in the constructor for ArrayAdapter/CustomAdapter, the code is passing the name of the layout view to be used for each item (R.layout.imageview_tutorial_layout) and the id of a textview widget in that layout (R.id.tutorialTextView)
- Now, set the listview's adapter through a call to: listview.setAdapter(adapter);
- To free up the image when views are recycled, implement RecycleListener callback as shown below
You can run the ImageViewTutorialActivity in Android Studio to try out the tutorial yourself.
Project Activities
Here are the activities you will work through in this project.
Simple Contact List
In this activity, you will build an app that shows a list of contacts as shown below. The list shows only 1 column/item (single item list)
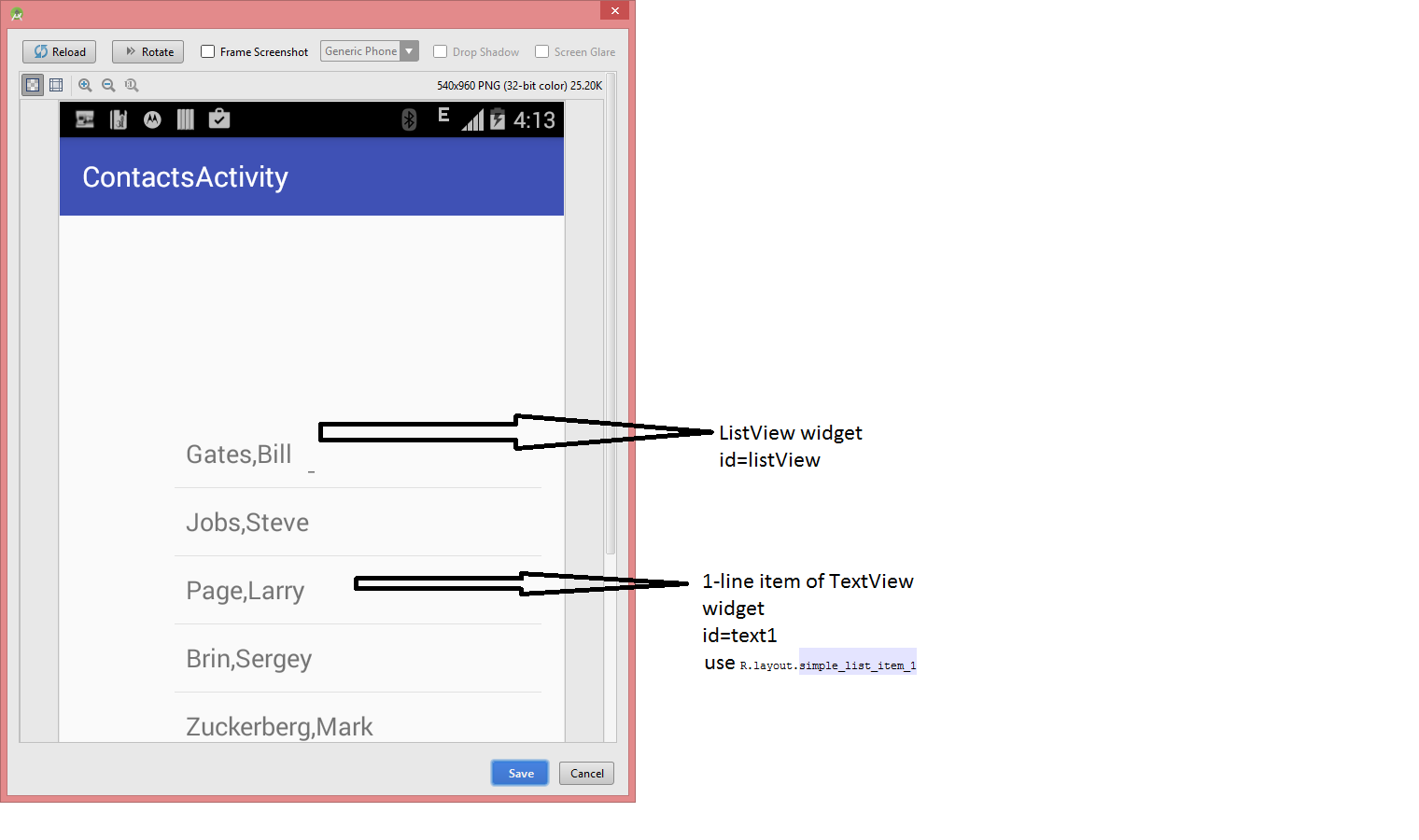
2 Item List Views
In this project, you will build an app that shows 2 items (fullname and company) for every row in the list. An example screenshot is shown below.
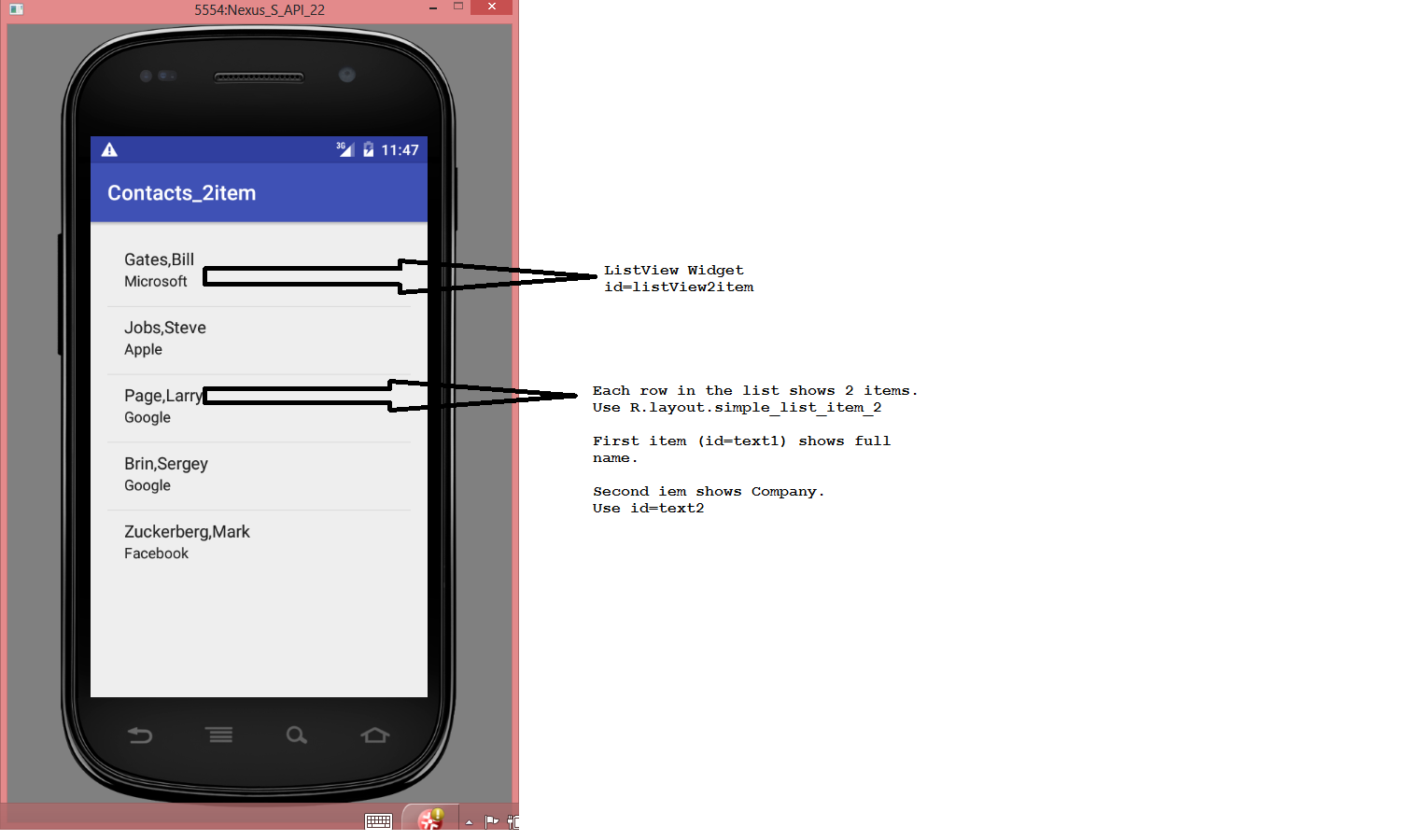
Sorting in ListViews
In ths project, you will enahnce the contact list app built in the previous step by adding 2 buttons to sort the list in ascending/descending order as shown below.
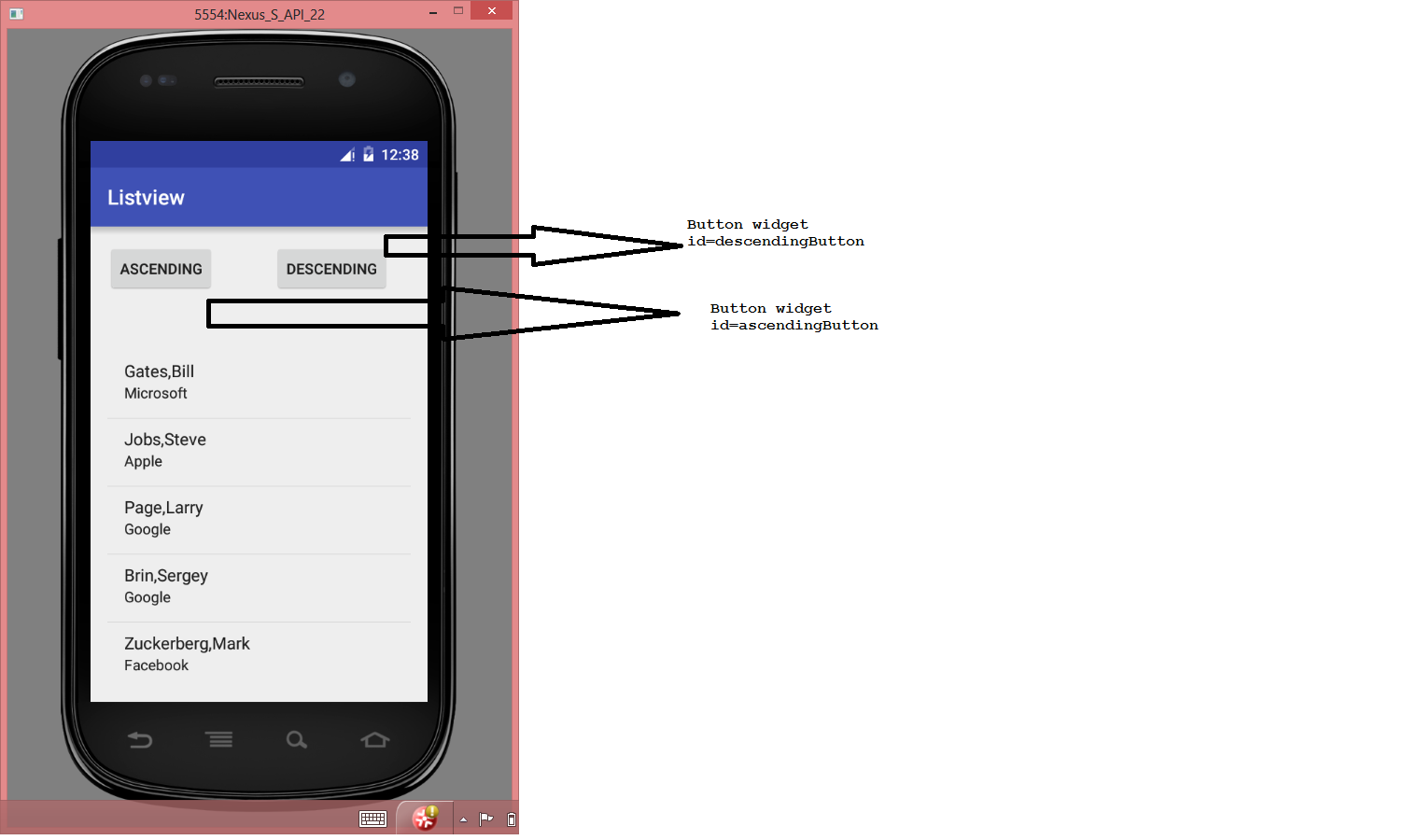
onClick of ascendingButton, you need to implement an onClickListener that will sort the contacts by FullName in ascending order as shown below.
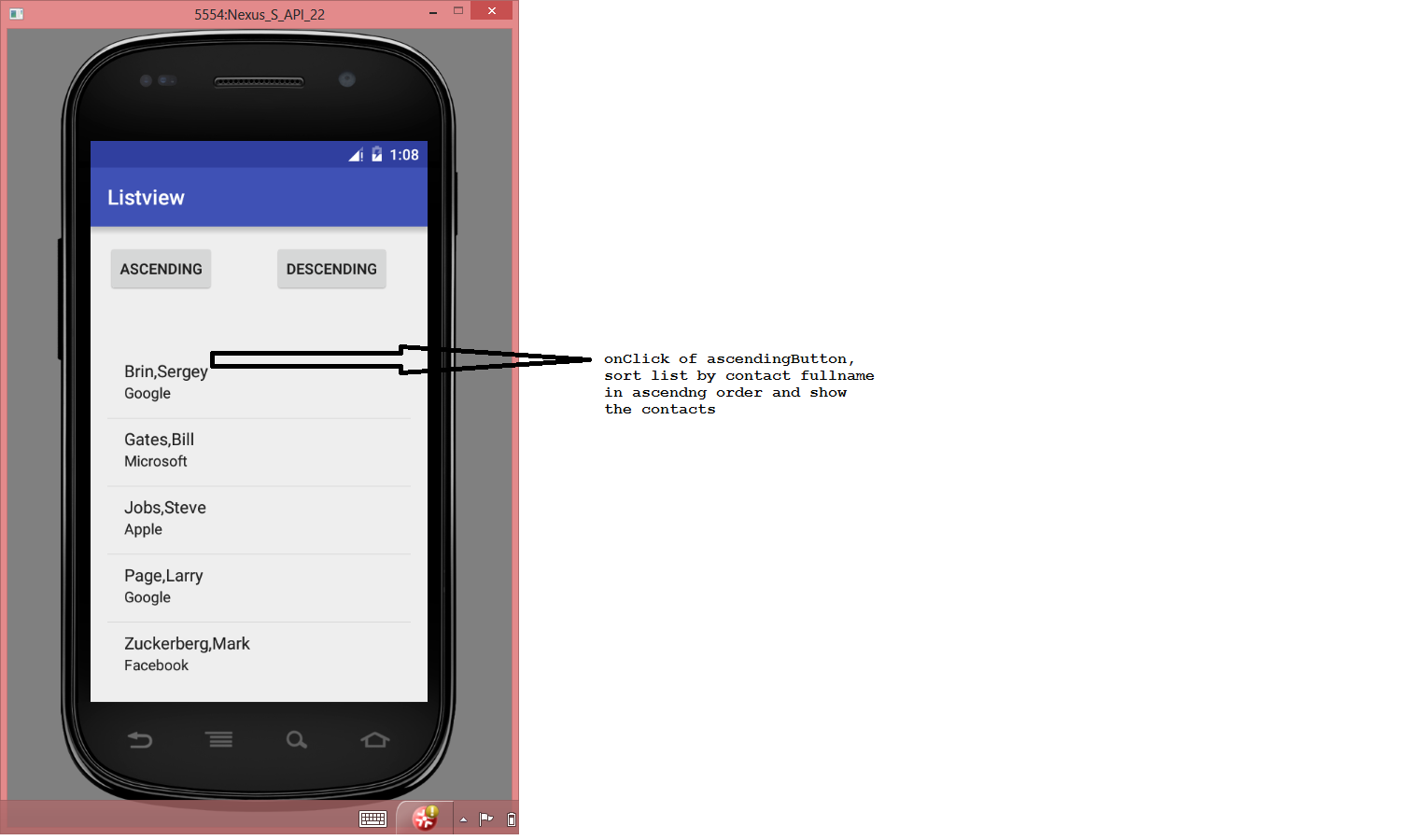
Similarly, onClick of descendingButton, sort and display the contacts list in descending order by FullName as shown below.
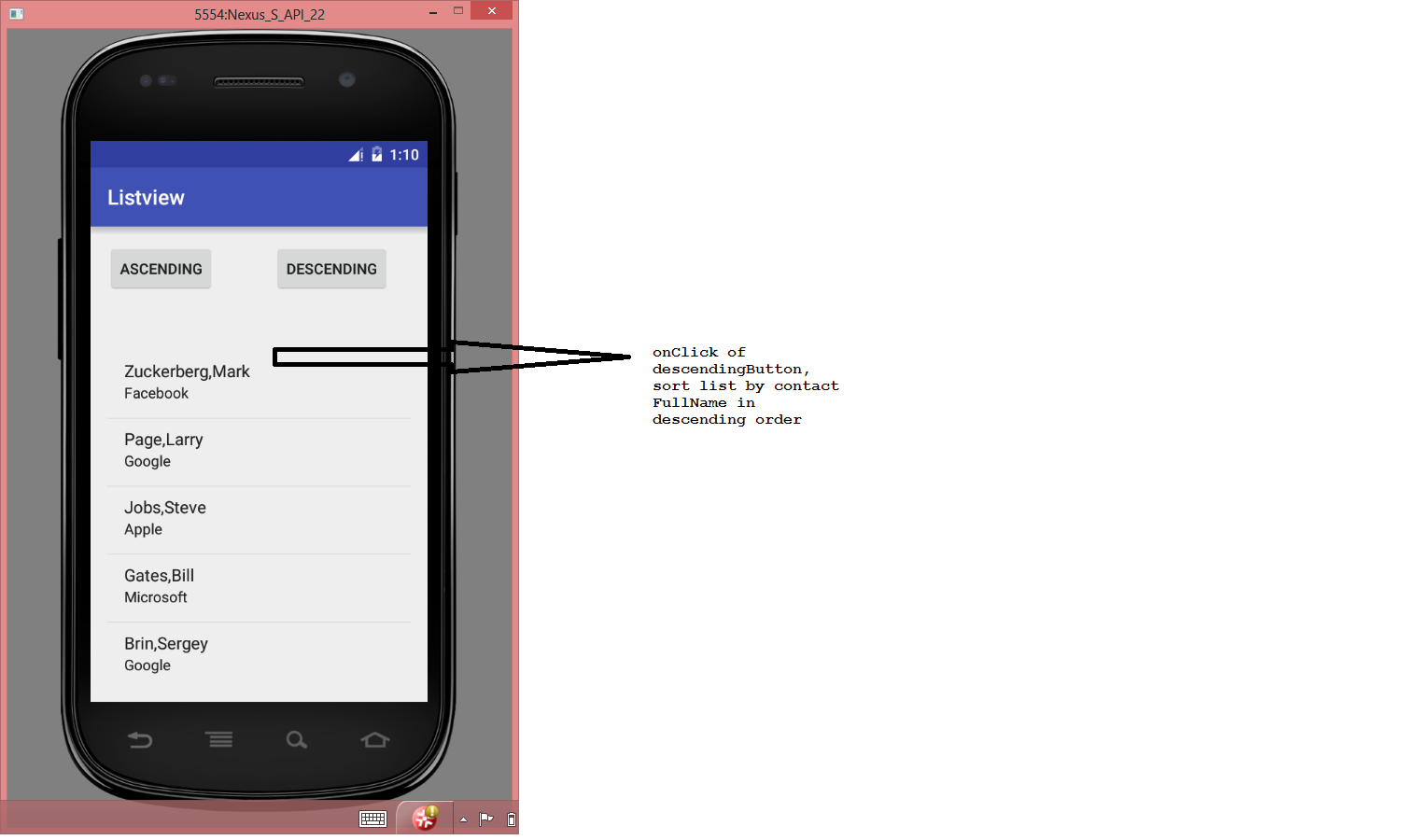
Pagination
When you have to deal with large amounts of data, a typical practice is to show a small number of items per page and provide a Next button to fetch more data. This approach is called pagination. In this project, you will implement pagination on a listview and display a list of countries.
A file containing country names is located at app\src\main\assets\countriesoftheworld.txt file. It contains 13 country names.
You have to build an app that shows a list view of 5 countries at a time as shown below.
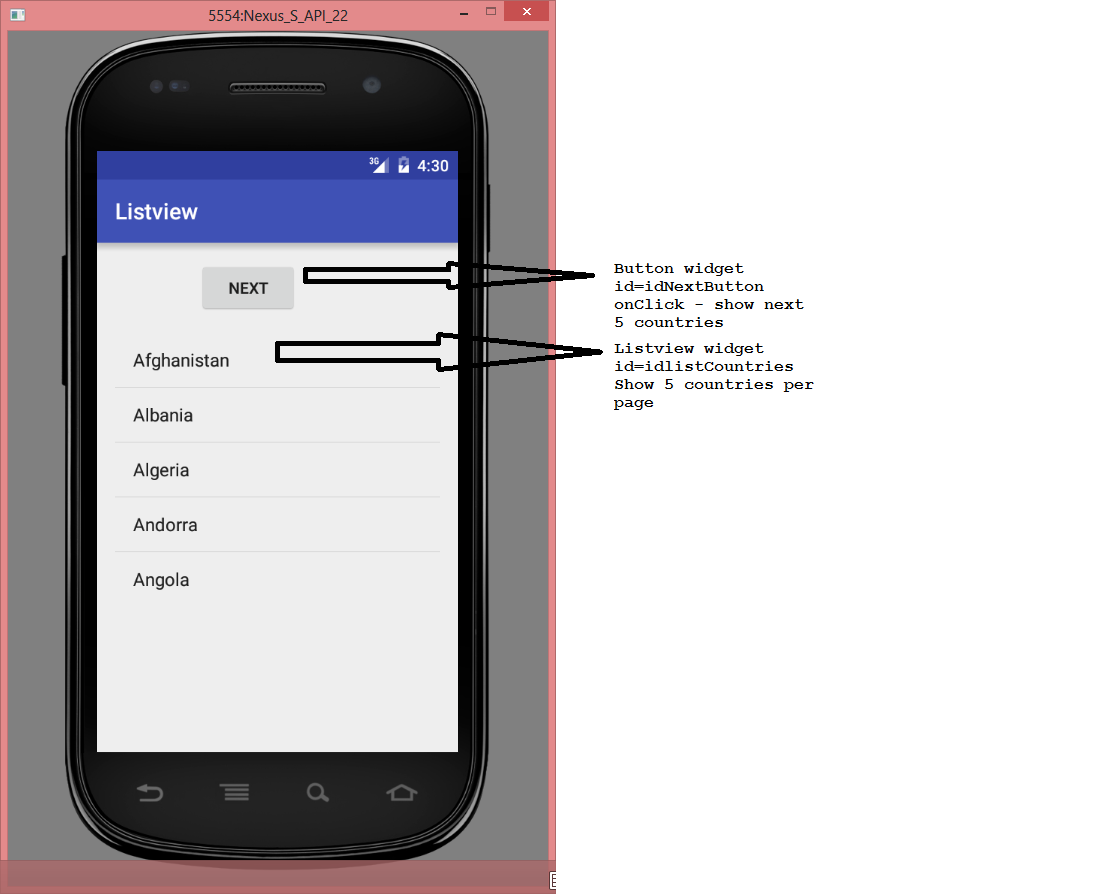
on click of the Next button, the app will fetch the next 5 countries and display them as shown below
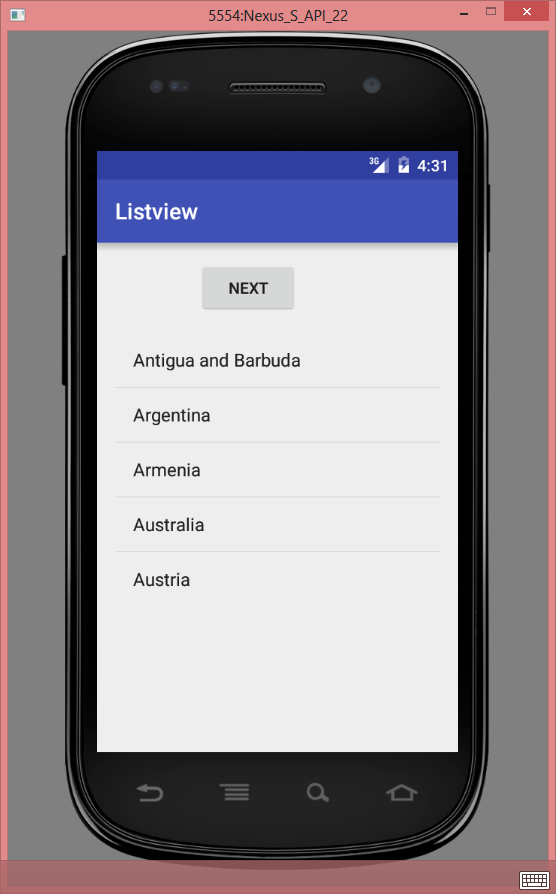
When the last page is reached, the next button should be disabled as shown below.
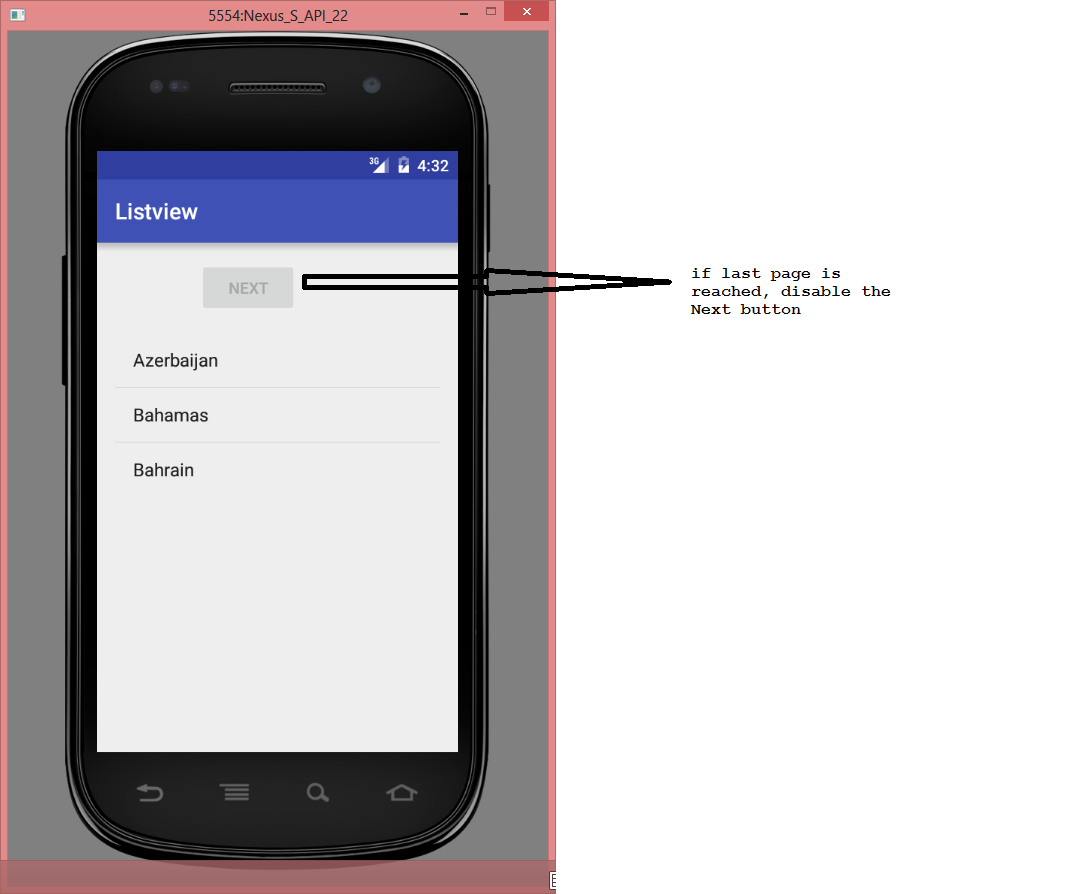
This Web Page was Built with PageBreeze Free HTML Editor